mirror of
https://git.mirrors.martin98.com/https://github.com/langgenius/dify.git
synced 2025-05-18 04:26:54 +08:00
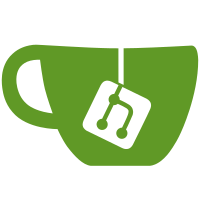
Co-authored-by: NFish <douxc512@gmail.com> Co-authored-by: zxhlyh <jasonapring2015@outlook.com> Co-authored-by: twwu <twwu@dify.ai> Co-authored-by: jZonG <jzongcode@gmail.com>
57 lines
1.6 KiB
TypeScript
57 lines
1.6 KiB
TypeScript
'use client'
|
|
|
|
import React from 'react'
|
|
import Button from '@/app/components/base/button'
|
|
import { useTranslation } from 'react-i18next'
|
|
|
|
type SetURLProps = {
|
|
repoUrl: string
|
|
onChange: (value: string) => void
|
|
onNext: () => void
|
|
onCancel: () => void
|
|
}
|
|
|
|
const SetURL: React.FC<SetURLProps> = ({ repoUrl, onChange, onNext, onCancel }) => {
|
|
const { t } = useTranslation()
|
|
return (
|
|
<>
|
|
<label
|
|
htmlFor='repoUrl'
|
|
className='flex flex-col items-start justify-center self-stretch text-text-secondary'
|
|
>
|
|
<span className='system-sm-semibold'>{t('plugin.installFromGitHub.gitHubRepo')}</span>
|
|
</label>
|
|
<input
|
|
type='url'
|
|
id='repoUrl'
|
|
name='repoUrl'
|
|
value={repoUrl}
|
|
onChange={e => onChange(e.target.value)}
|
|
className='shadows-shadow-xs system-sm-regular flex grow items-center gap-[2px]
|
|
self-stretch overflow-hidden text-ellipsis rounded-lg border border-components-input-border-active
|
|
bg-components-input-bg-active p-2 text-components-input-text-filled'
|
|
placeholder='Please enter GitHub repo URL'
|
|
/>
|
|
<div className='mt-4 flex items-center justify-end gap-2 self-stretch'>
|
|
<Button
|
|
variant='secondary'
|
|
className='min-w-[72px]'
|
|
onClick={onCancel}
|
|
>
|
|
{t('plugin.installModal.cancel')}
|
|
</Button>
|
|
<Button
|
|
variant='primary'
|
|
className='min-w-[72px]'
|
|
onClick={onNext}
|
|
disabled={!repoUrl.trim()}
|
|
>
|
|
{t('plugin.installModal.next')}
|
|
</Button>
|
|
</div>
|
|
</>
|
|
)
|
|
}
|
|
|
|
export default SetURL
|