mirror of
https://git.mirrors.martin98.com/https://github.com/langgenius/dify.git
synced 2025-05-24 23:28:32 +08:00
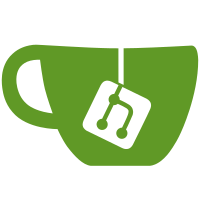
Signed-off-by: -LAN- <laipz8200@outlook.com> Co-authored-by: Hash Brown <hi@xzd.me> Co-authored-by: crazywoola <427733928@qq.com> Co-authored-by: GareArc <chen4851@purdue.edu> Co-authored-by: Byron.wang <byron@dify.ai> Co-authored-by: Joel <iamjoel007@gmail.com> Co-authored-by: -LAN- <laipz8200@outlook.com> Co-authored-by: Garfield Dai <dai.hai@foxmail.com> Co-authored-by: KVOJJJin <jzongcode@gmail.com> Co-authored-by: Alexi.F <654973939@qq.com> Co-authored-by: Xiyuan Chen <52963600+GareArc@users.noreply.github.com> Co-authored-by: kautsar_masuara <61046989+izon-masuara@users.noreply.github.com> Co-authored-by: achmad-kautsar <achmad.kautsar@insignia.co.id> Co-authored-by: Xin Zhang <sjhpzx@gmail.com> Co-authored-by: kelvintsim <83445753+kelvintsim@users.noreply.github.com> Co-authored-by: zxhlyh <jasonapring2015@outlook.com> Co-authored-by: Zixuan Cheng <61724187+Theysua@users.noreply.github.com>
88 lines
2.7 KiB
Python
88 lines
2.7 KiB
Python
from functools import wraps
|
|
|
|
from flask_login import current_user
|
|
from flask_restful import Resource
|
|
from werkzeug.exceptions import NotFound
|
|
|
|
from controllers.console.explore.error import AppAccessDeniedError
|
|
from controllers.console.wraps import account_initialization_required
|
|
from extensions.ext_database import db
|
|
from libs.login import login_required
|
|
from models import InstalledApp
|
|
from services.app_service import AppService
|
|
from services.enterprise.enterprise_service import EnterpriseService
|
|
from services.feature_service import FeatureService
|
|
|
|
|
|
def installed_app_required(view=None):
|
|
def decorator(view):
|
|
@wraps(view)
|
|
def decorated(*args, **kwargs):
|
|
if not kwargs.get("installed_app_id"):
|
|
raise ValueError("missing installed_app_id in path parameters")
|
|
|
|
installed_app_id = kwargs.get("installed_app_id")
|
|
installed_app_id = str(installed_app_id)
|
|
|
|
del kwargs["installed_app_id"]
|
|
|
|
installed_app = (
|
|
db.session.query(InstalledApp)
|
|
.filter(
|
|
InstalledApp.id == str(installed_app_id), InstalledApp.tenant_id == current_user.current_tenant_id
|
|
)
|
|
.first()
|
|
)
|
|
|
|
if installed_app is None:
|
|
raise NotFound("Installed app not found")
|
|
|
|
if not installed_app.app:
|
|
db.session.delete(installed_app)
|
|
db.session.commit()
|
|
|
|
raise NotFound("Installed app not found")
|
|
|
|
return view(installed_app, *args, **kwargs)
|
|
|
|
return decorated
|
|
|
|
if view:
|
|
return decorator(view)
|
|
return decorator
|
|
|
|
|
|
def user_allowed_to_access_app(view=None):
|
|
def decorator(view):
|
|
@wraps(view)
|
|
def decorated(installed_app: InstalledApp, *args, **kwargs):
|
|
feature = FeatureService.get_system_features()
|
|
if feature.webapp_auth.enabled:
|
|
app_id = installed_app.app_id
|
|
app_code = AppService.get_app_code_by_id(app_id)
|
|
res = EnterpriseService.WebAppAuth.is_user_allowed_to_access_webapp(
|
|
user_id=str(current_user.id),
|
|
app_code=app_code,
|
|
)
|
|
if not res:
|
|
raise AppAccessDeniedError()
|
|
|
|
return view(installed_app, *args, **kwargs)
|
|
|
|
return decorated
|
|
|
|
if view:
|
|
return decorator(view)
|
|
return decorator
|
|
|
|
|
|
class InstalledAppResource(Resource):
|
|
# must be reversed if there are multiple decorators
|
|
|
|
method_decorators = [
|
|
user_allowed_to_access_app,
|
|
installed_app_required,
|
|
account_initialization_required,
|
|
login_required,
|
|
]
|