mirror of
https://git.mirrors.martin98.com/https://github.com/langgenius/dify.git
synced 2025-05-29 17:45:12 +08:00
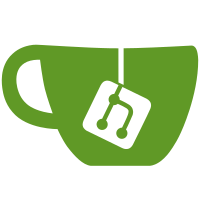
Co-authored-by: NFish <douxc512@gmail.com> Co-authored-by: zxhlyh <jasonapring2015@outlook.com> Co-authored-by: twwu <twwu@dify.ai> Co-authored-by: jZonG <jzongcode@gmail.com>
51 lines
1.5 KiB
TypeScript
51 lines
1.5 KiB
TypeScript
'use client'
|
|
import React, { useState } from 'react'
|
|
import StrategyDetailPanel from './strategy-detail'
|
|
import type {
|
|
StrategyDetail,
|
|
} from '@/app/components/plugins/types'
|
|
import type { Locale } from '@/i18n'
|
|
import { useRenderI18nObject } from '@/hooks/use-i18n'
|
|
import cn from '@/utils/classnames'
|
|
|
|
type Props = {
|
|
provider: {
|
|
author: string
|
|
name: string
|
|
description: Record<Locale, string>
|
|
tenant_id: string
|
|
icon: string
|
|
label: Record<Locale, string>
|
|
tags: string[]
|
|
}
|
|
detail: StrategyDetail
|
|
}
|
|
|
|
const StrategyItem = ({
|
|
provider,
|
|
detail,
|
|
}: Props) => {
|
|
const getValueFromI18nObject = useRenderI18nObject()
|
|
const [showDetail, setShowDetail] = useState(false)
|
|
|
|
return (
|
|
<>
|
|
<div
|
|
className={cn('bg-components-panel-item-bg mb-2 cursor-pointer rounded-xl border-[0.5px] border-components-panel-border-subtle px-4 py-3 shadow-xs hover:bg-components-panel-on-panel-item-bg-hover')}
|
|
onClick={() => setShowDetail(true)}
|
|
>
|
|
<div className='system-md-semibold pb-0.5 text-text-secondary'>{getValueFromI18nObject(detail.identity.label)}</div>
|
|
<div className='system-xs-regular line-clamp-2 text-text-tertiary' title={getValueFromI18nObject(detail.description)}>{getValueFromI18nObject(detail.description)}</div>
|
|
</div>
|
|
{showDetail && (
|
|
<StrategyDetailPanel
|
|
provider={provider}
|
|
detail={detail}
|
|
onHide={() => setShowDetail(false)}
|
|
/>
|
|
)}
|
|
</>
|
|
)
|
|
}
|
|
export default StrategyItem
|