mirror of
https://git.mirrors.martin98.com/https://github.com/langgenius/dify.git
synced 2025-07-05 22:15:09 +08:00
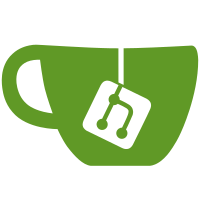
Co-authored-by: NFish <douxc512@gmail.com> Co-authored-by: zxhlyh <jasonapring2015@outlook.com> Co-authored-by: twwu <twwu@dify.ai> Co-authored-by: jZonG <jzongcode@gmail.com>
43 lines
1.3 KiB
TypeScript
43 lines
1.3 KiB
TypeScript
import { useCallback } from 'react'
|
|
import type { ChangeEvent } from 'react'
|
|
import { useTranslation } from 'react-i18next'
|
|
import { RiCloseCircleFill, RiSearchLine } from '@remixicon/react'
|
|
import cn from '@/utils/classnames'
|
|
|
|
type SearchInputProps = {
|
|
value: string
|
|
onChange: (v: string) => void
|
|
}
|
|
const SearchInput = ({
|
|
value,
|
|
onChange,
|
|
}: SearchInputProps) => {
|
|
const { t } = useTranslation()
|
|
|
|
const handleClear = useCallback(() => {
|
|
onChange('')
|
|
}, [onChange])
|
|
|
|
return (
|
|
<div className={cn('flex h-8 w-[200px] items-center rounded-lg bg-components-input-bg-normal p-2')}>
|
|
<RiSearchLine className={'mr-0.5 h-4 w-4 shrink-0 text-components-input-text-placeholder'} />
|
|
<input
|
|
className='min-w-0 grow appearance-none border-0 bg-transparent px-1 text-[13px] leading-[16px] text-components-input-text-filled outline-0 placeholder:text-components-input-text-placeholder'
|
|
value={value}
|
|
onChange={(e: ChangeEvent<HTMLInputElement>) => onChange(e.target.value)}
|
|
placeholder={t('common.dataSource.notion.selector.searchPages') || ''}
|
|
/>
|
|
{
|
|
value && (
|
|
<RiCloseCircleFill
|
|
className={'h-4 w-4 shrink-0 cursor-pointer text-components-input-text-placeholder'}
|
|
onClick={handleClear}
|
|
/>
|
|
)
|
|
}
|
|
</div>
|
|
)
|
|
}
|
|
|
|
export default SearchInput
|