mirror of
https://git.mirrors.martin98.com/https://github.com/infiniflow/ragflow.git
synced 2025-07-16 13:01:50 +08:00
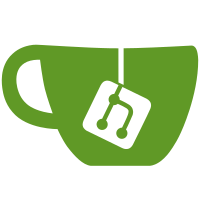
### What problem does this PR solve? feat: Send message with uuid #2088 ### Type of change - [x] New Feature (non-breaking change which adds functionality)
26 lines
792 B
TypeScript
26 lines
792 B
TypeScript
import { EmptyConversationId, MessageType } from '@/constants/chat';
|
|
import { Message } from '@/interfaces/database/chat';
|
|
import { IMessage } from '@/pages/chat/interface';
|
|
import { v4 as uuid } from 'uuid';
|
|
|
|
export const isConversationIdExist = (conversationId: string) => {
|
|
return conversationId !== EmptyConversationId && conversationId !== '';
|
|
};
|
|
|
|
export const buildMessageUuid = (message: Partial<Message | IMessage>) => {
|
|
if ('id' in message && message.id) {
|
|
return message.role === MessageType.User
|
|
? `${MessageType.User}_${message.id}`
|
|
: `${MessageType.Assistant}_${message.id}`;
|
|
}
|
|
return uuid();
|
|
};
|
|
|
|
export const getMessagePureId = (id: string) => {
|
|
const strings = id.split('_');
|
|
if (strings.length > 0) {
|
|
return strings.at(-1);
|
|
}
|
|
return id;
|
|
};
|