mirror of
https://git.mirrors.martin98.com/https://github.com/infiniflow/ragflow.git
synced 2025-05-25 15:48:07 +08:00
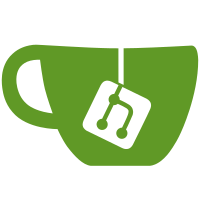
### What problem does this PR solve? feat: create folder feat: ensure that all files in the current folder can be correctly requested after renaming the folder #345 ### Type of change - [x] New Feature (non-breaking change which adds functionality)
97 lines
2.1 KiB
TypeScript
97 lines
2.1 KiB
TypeScript
import { IFileListRequestBody } from '@/interfaces/request/file-manager';
|
|
import { useCallback } from 'react';
|
|
import { useDispatch, useSelector } from 'umi';
|
|
|
|
export const useFetchFileList = () => {
|
|
const dispatch = useDispatch();
|
|
|
|
const fetchFileList = useCallback(
|
|
(payload: IFileListRequestBody) => {
|
|
return dispatch<any>({
|
|
type: 'fileManager/listFile',
|
|
payload,
|
|
});
|
|
},
|
|
[dispatch],
|
|
);
|
|
|
|
return fetchFileList;
|
|
};
|
|
|
|
export const useRemoveFile = () => {
|
|
const dispatch = useDispatch();
|
|
|
|
const removeFile = useCallback(
|
|
(fileIds: string[], parentId: string) => {
|
|
return dispatch<any>({
|
|
type: 'fileManager/removeFile',
|
|
payload: { fileIds, parentId },
|
|
});
|
|
},
|
|
[dispatch],
|
|
);
|
|
|
|
return removeFile;
|
|
};
|
|
|
|
export const useRenameFile = () => {
|
|
const dispatch = useDispatch();
|
|
|
|
const renameFile = useCallback(
|
|
(fileId: string, name: string, parentId: string) => {
|
|
return dispatch<any>({
|
|
type: 'fileManager/renameFile',
|
|
payload: { fileId, name, parentId },
|
|
});
|
|
},
|
|
[dispatch],
|
|
);
|
|
|
|
return renameFile;
|
|
};
|
|
|
|
export const useFetchParentFolderList = () => {
|
|
const dispatch = useDispatch();
|
|
|
|
const fetchParentFolderList = useCallback(
|
|
(fileId: string) => {
|
|
return dispatch<any>({
|
|
type: 'fileManager/getAllParentFolder',
|
|
payload: { fileId },
|
|
});
|
|
},
|
|
[dispatch],
|
|
);
|
|
|
|
return fetchParentFolderList;
|
|
};
|
|
|
|
export const useCreateFolder = () => {
|
|
const dispatch = useDispatch();
|
|
|
|
const createFolder = useCallback(
|
|
(parentId: string, name: string) => {
|
|
return dispatch<any>({
|
|
type: 'fileManager/createFolder',
|
|
payload: { parentId, name, type: 'folder' },
|
|
});
|
|
},
|
|
[dispatch],
|
|
);
|
|
|
|
return createFolder;
|
|
};
|
|
|
|
export const useSelectFileList = () => {
|
|
const fileList = useSelector((state) => state.fileManager.fileList);
|
|
|
|
return fileList;
|
|
};
|
|
|
|
export const useSelectParentFolderList = () => {
|
|
const parentFolderList = useSelector(
|
|
(state) => state.fileManager.parentFolderList,
|
|
);
|
|
return parentFolderList.toReversed();
|
|
};
|