mirror of
https://git.mirrors.martin98.com/https://github.com/infiniflow/ragflow.git
synced 2025-04-23 22:50:17 +08:00
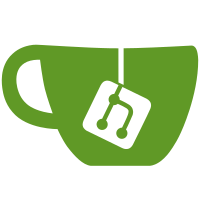
### What problem does this PR solve? Langfuse update model has no fields attribute ### Type of change - [x] Bug Fix (non-breaking change which fixes an issue)
58 lines
2.0 KiB
Python
58 lines
2.0 KiB
Python
#
|
|
# Copyright 2025 The InfiniFlow Authors. All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
#
|
|
|
|
from datetime import datetime
|
|
|
|
import peewee
|
|
|
|
from api.db.db_models import DB, TenantLangfuse
|
|
from api.db.services.common_service import CommonService
|
|
from api.utils import current_timestamp, datetime_format
|
|
|
|
|
|
class TenantLangfuseService(CommonService):
|
|
"""
|
|
All methods that modify the status should be enclosed within a DB.atomic() context to ensure atomicity
|
|
and maintain data integrity in case of errors during execution.
|
|
"""
|
|
|
|
model = TenantLangfuse
|
|
|
|
@classmethod
|
|
@DB.connection_context()
|
|
def filter_by_tenant(cls, tenant_id):
|
|
fields = [cls.model.host, cls.model.secret_key, cls.model.public_key]
|
|
try:
|
|
keys = cls.model.select(*fields).where(cls.model.tenant_id == tenant_id).first()
|
|
return keys
|
|
except peewee.DoesNotExist:
|
|
return None
|
|
|
|
@classmethod
|
|
def update_by_tenant(cls, tenant_id, langfuse_keys):
|
|
langfuse_keys["update_time"] = current_timestamp()
|
|
langfuse_keys["update_date"] = datetime_format(datetime.now())
|
|
return cls.model.update(**langfuse_keys).where(cls.model.tenant_id == tenant_id).execute()
|
|
|
|
@classmethod
|
|
def save(cls, **kwargs):
|
|
kwargs["create_time"] = current_timestamp()
|
|
kwargs["create_date"] = datetime_format(datetime.now())
|
|
kwargs["update_time"] = current_timestamp()
|
|
kwargs["update_date"] = datetime_format(datetime.now())
|
|
obj = cls.model.create(**kwargs)
|
|
return obj
|