mirror of
https://git.mirrors.martin98.com/https://github.com/infiniflow/ragflow.git
synced 2025-07-16 07:01:45 +08:00
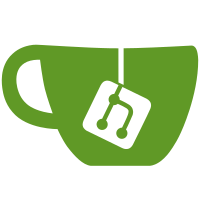
### What problem does this PR solve? Test cases about dataset ### Type of change - [x] Other (please describe): test cases --------- Signed-off-by: jinhai <haijin.chn@gmail.com>
35 lines
1.0 KiB
Python
35 lines
1.0 KiB
Python
import os
|
|
import requests
|
|
|
|
HOST_ADDRESS = os.getenv('HOST_ADDRESS', 'http://127.0.0.1:9380')
|
|
|
|
DATASET_NAME_LIMIT = 128
|
|
|
|
def create_dataset(auth, dataset_name):
|
|
authorization = {"Authorization": auth}
|
|
url = f"{HOST_ADDRESS}/v1/kb/create"
|
|
json = {"name": dataset_name}
|
|
res = requests.post(url=url, headers=authorization, json=json)
|
|
return res.json()
|
|
|
|
|
|
def list_dataset(auth, page_number):
|
|
authorization = {"Authorization": auth}
|
|
url = f"{HOST_ADDRESS}/v1/kb/list?page={page_number}"
|
|
res = requests.get(url=url, headers=authorization)
|
|
return res.json()
|
|
|
|
|
|
def rm_dataset(auth, dataset_id):
|
|
authorization = {"Authorization": auth}
|
|
url = f"{HOST_ADDRESS}/v1/kb/rm"
|
|
json = {"kb_id": dataset_id}
|
|
res = requests.post(url=url, headers=authorization, json=json)
|
|
return res.json()
|
|
|
|
def update_dataset(auth, json_req):
|
|
authorization = {"Authorization": auth}
|
|
url = f"{HOST_ADDRESS}/v1/kb/update"
|
|
res = requests.post(url=url, headers=authorization, json=json_req)
|
|
return res.json()
|