mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-05-10 05:19:02 +08:00
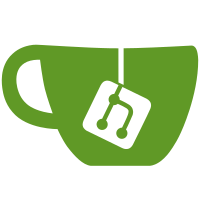
The button was opening the online Marketplace, which was wrong. This fix changes the behavior to open the in-Cura Marketplace at the materials tab. In order to achieve that, the button calls an action called materialsMarketplace, which in turn is connected through a Connection in the ApplicationMenu. When the action is triggered, it first launched the Toolbox and then it sets its category to the materials tab. Since the callExtensionMethod does not allow us to provide input arguments to the called method, a new method had to be created in the toolbox that changes the view to the materials tab, instead of immediately calling the setViewCategory("material"). CURA-7027
177 lines
5.5 KiB
QML
177 lines
5.5 KiB
QML
// Copyright (c) 2018 Ultimaker B.V.
|
|
// Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
import QtQuick 2.7
|
|
import QtQuick.Controls 1.4
|
|
import QtQuick.Controls.Styles 1.4
|
|
import QtQuick.Layouts 1.1
|
|
import QtQuick.Dialogs 1.2
|
|
|
|
import UM 1.3 as UM
|
|
import Cura 1.1 as Cura
|
|
|
|
import "../Menus"
|
|
import "../Dialogs"
|
|
|
|
Item
|
|
{
|
|
id: menu
|
|
width: applicationMenu.width
|
|
height: applicationMenu.height
|
|
property alias window: applicationMenu.window
|
|
|
|
UM.ApplicationMenu
|
|
{
|
|
id: applicationMenu
|
|
|
|
FileMenu { title: catalog.i18nc("@title:menu menubar:toplevel", "&File") }
|
|
|
|
Menu
|
|
{
|
|
title: catalog.i18nc("@title:menu menubar:toplevel", "&Edit")
|
|
|
|
MenuItem { action: Cura.Actions.undo }
|
|
MenuItem { action: Cura.Actions.redo }
|
|
MenuSeparator { }
|
|
MenuItem { action: Cura.Actions.selectAll }
|
|
MenuItem { action: Cura.Actions.arrangeAll }
|
|
MenuItem { action: Cura.Actions.deleteSelection }
|
|
MenuItem { action: Cura.Actions.deleteAll }
|
|
MenuItem { action: Cura.Actions.resetAllTranslation }
|
|
MenuItem { action: Cura.Actions.resetAll }
|
|
MenuSeparator { }
|
|
MenuItem { action: Cura.Actions.groupObjects }
|
|
MenuItem { action: Cura.Actions.mergeObjects }
|
|
MenuItem { action: Cura.Actions.unGroupObjects }
|
|
}
|
|
|
|
ViewMenu { title: catalog.i18nc("@title:menu menubar:toplevel", "&View") }
|
|
|
|
SettingsMenu { title: catalog.i18nc("@title:menu menubar:toplevel", "&Settings") }
|
|
|
|
Menu
|
|
{
|
|
id: extensionMenu
|
|
title: catalog.i18nc("@title:menu menubar:toplevel", "E&xtensions")
|
|
|
|
Instantiator
|
|
{
|
|
id: extensions
|
|
model: UM.ExtensionModel { }
|
|
|
|
Menu
|
|
{
|
|
id: sub_menu
|
|
title: model.name;
|
|
visible: actions != null
|
|
enabled: actions != null
|
|
Instantiator
|
|
{
|
|
model: actions
|
|
MenuItem
|
|
{
|
|
text: model.text
|
|
onTriggered: extensions.model.subMenuTriggered(name, model.text)
|
|
}
|
|
onObjectAdded: sub_menu.insertItem(index, object)
|
|
onObjectRemoved: sub_menu.removeItem(object)
|
|
}
|
|
}
|
|
|
|
onObjectAdded: extensionMenu.insertItem(index, object)
|
|
onObjectRemoved: extensionMenu.removeItem(object)
|
|
}
|
|
}
|
|
|
|
Menu
|
|
{
|
|
id: preferencesMenu
|
|
title: catalog.i18nc("@title:menu menubar:toplevel", "P&references")
|
|
|
|
MenuItem { action: Cura.Actions.preferences }
|
|
}
|
|
|
|
Menu
|
|
{
|
|
id: helpMenu
|
|
title: catalog.i18nc("@title:menu menubar:toplevel", "&Help")
|
|
|
|
MenuItem { action: Cura.Actions.showProfileFolder }
|
|
MenuItem { action: Cura.Actions.showTroubleshooting}
|
|
MenuItem { action: Cura.Actions.documentation }
|
|
MenuItem { action: Cura.Actions.reportBug }
|
|
MenuSeparator { }
|
|
MenuItem { action: Cura.Actions.whatsNew }
|
|
MenuItem { action: Cura.Actions.about }
|
|
}
|
|
}
|
|
|
|
// ###############################################################################################
|
|
// Definition of other components that are linked to the menus
|
|
// ###############################################################################################
|
|
|
|
WorkspaceSummaryDialog
|
|
{
|
|
id: saveWorkspaceDialog
|
|
property var args
|
|
onYes: UM.OutputDeviceManager.requestWriteToDevice("local_file", PrintInformation.jobName, args)
|
|
}
|
|
|
|
MessageDialog
|
|
{
|
|
id: newProjectDialog
|
|
modality: Qt.ApplicationModal
|
|
title: catalog.i18nc("@title:window", "New project")
|
|
text: catalog.i18nc("@info:question", "Are you sure you want to start a new project? This will clear the build plate and any unsaved settings.")
|
|
standardButtons: StandardButton.Yes | StandardButton.No
|
|
icon: StandardIcon.Question
|
|
onYes:
|
|
{
|
|
CuraApplication.deleteAll();
|
|
Cura.Actions.resetProfile.trigger();
|
|
UM.Controller.setActiveStage("PrepareStage")
|
|
}
|
|
}
|
|
|
|
UM.ExtensionModel
|
|
{
|
|
id: curaExtensions
|
|
}
|
|
|
|
// ###############################################################################################
|
|
// Definition of all the connections
|
|
// ###############################################################################################
|
|
|
|
Connections
|
|
{
|
|
target: Cura.Actions.newProject
|
|
onTriggered:
|
|
{
|
|
if(Printer.platformActivity || Cura.MachineManager.hasUserSettings)
|
|
{
|
|
newProjectDialog.visible = true
|
|
}
|
|
}
|
|
}
|
|
|
|
// show the plugin browser dialog
|
|
Connections
|
|
{
|
|
target: Cura.Actions.browsePackages
|
|
onTriggered:
|
|
{
|
|
curaExtensions.callExtensionMethod("Toolbox", "launch")
|
|
}
|
|
}
|
|
|
|
// Show the Marketplace dialog at the materials tab
|
|
Connections
|
|
{
|
|
target: Cura.Actions.marketplaceMaterials
|
|
onTriggered:
|
|
{
|
|
curaExtensions.callExtensionMethod("Toolbox", "launch")
|
|
curaExtensions.callExtensionMethod("Toolbox", "setViewCategoryToMaterials")
|
|
}
|
|
}
|
|
} |