mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-05-03 09:14:24 +08:00
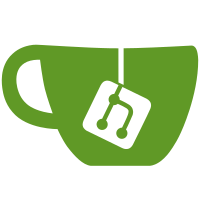
After a lot of discussion and finding out what the hell was going on, we figured out we made a pretty big derp by only setting a single connection_type in the metadata of the machine. What it's actually doing is describing what connection types have been configured (and not just randomly displaying whatever output device set the value last)
63 lines
2.5 KiB
Python
63 lines
2.5 KiB
Python
# Copyright (c) 2018 Ultimaker B.V.
|
|
# Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
from UM.Qt.ListModel import ListModel
|
|
|
|
from PyQt5.QtCore import pyqtProperty, Qt, pyqtSignal
|
|
|
|
from cura.PrinterOutputDevice import ConnectionType
|
|
from cura.Settings.CuraContainerRegistry import CuraContainerRegistry
|
|
|
|
from cura.Settings.GlobalStack import GlobalStack
|
|
|
|
|
|
class GlobalStacksModel(ListModel):
|
|
NameRole = Qt.UserRole + 1
|
|
IdRole = Qt.UserRole + 2
|
|
HasRemoteConnectionRole = Qt.UserRole + 3
|
|
ConnectionTypeRole = Qt.UserRole + 4
|
|
MetaDataRole = Qt.UserRole + 5
|
|
|
|
def __init__(self, parent = None):
|
|
super().__init__(parent)
|
|
self.addRoleName(self.NameRole, "name")
|
|
self.addRoleName(self.IdRole, "id")
|
|
self.addRoleName(self.HasRemoteConnectionRole, "hasRemoteConnection")
|
|
self.addRoleName(self.MetaDataRole, "metadata")
|
|
self._container_stacks = []
|
|
|
|
# Listen to changes
|
|
CuraContainerRegistry.getInstance().containerAdded.connect(self._onContainerChanged)
|
|
CuraContainerRegistry.getInstance().containerMetaDataChanged.connect(self._onContainerChanged)
|
|
CuraContainerRegistry.getInstance().containerRemoved.connect(self._onContainerChanged)
|
|
self._filter_dict = {}
|
|
self._update()
|
|
|
|
## Handler for container added/removed events from registry
|
|
def _onContainerChanged(self, container):
|
|
# We only need to update when the added / removed container GlobalStack
|
|
if isinstance(container, GlobalStack):
|
|
self._update()
|
|
|
|
def _update(self) -> None:
|
|
items = []
|
|
|
|
container_stacks = CuraContainerRegistry.getInstance().findContainerStacks(type = "machine")
|
|
|
|
for container_stack in container_stacks:
|
|
has_remote_connection = False
|
|
|
|
for connection_type in container_stack.configuredConnectionTypes:
|
|
has_remote_connection |= connection_type in [ConnectionType.NetworkConnection.value, ConnectionType.CloudConnection.value]
|
|
|
|
if container_stack.getMetaDataEntry("hidden", False) in ["True", True]:
|
|
continue
|
|
|
|
# TODO: Remove reference to connect group name.
|
|
items.append({"name": container_stack.getMetaDataEntry("connect_group_name", container_stack.getName()),
|
|
"id": container_stack.getId(),
|
|
"hasRemoteConnection": has_remote_connection,
|
|
"metadata": container_stack.getMetaData().copy()})
|
|
items.sort(key=lambda i: not i["hasRemoteConnection"])
|
|
self.setItems(items)
|