mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-02 21:25:13 +08:00
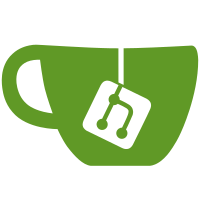
The profile name in the translation string was hard coded to be prepended before the actual string. Put this in the translation string as some languages may change the position of this part of the sentence.
106 lines
3.1 KiB
QML
106 lines
3.1 KiB
QML
import QtQuick 2.10
|
|
|
|
import UM 1.6 as UM
|
|
import Cura 1.6 as Cura
|
|
|
|
Item
|
|
{
|
|
height: visible ? UM.Theme.getSize("action_button_icon").height : 0
|
|
visible: Cura.SimpleModeSettingsManager.isProfileCustomized || Cura.MachineManager.hasCustomQuality
|
|
|
|
Rectangle
|
|
{
|
|
id: warningIcon
|
|
color: UM.Theme.getColor("um_yellow_5")
|
|
height: UM.Theme.getSize("action_button_icon").height
|
|
width: height
|
|
radius: width
|
|
anchors
|
|
{
|
|
left: parent.left
|
|
verticalCenter: parent.verticalCenter
|
|
}
|
|
UM.ColorImage
|
|
{
|
|
height: UM.Theme.getSize("action_button_icon").height
|
|
width: height
|
|
source: UM.Theme.getIcon("Warning", "low")
|
|
}
|
|
}
|
|
|
|
UM.Label
|
|
{
|
|
id: warning
|
|
width: parent.width - warningIcon.width - resetToDefaultQualityButton.width
|
|
anchors
|
|
{
|
|
left: warningIcon.right
|
|
verticalCenter: parent.verticalCenter
|
|
leftMargin: UM.Theme.getSize("thin_margin").width
|
|
}
|
|
|
|
wrapMode: Text.WordWrap
|
|
|
|
states: [
|
|
State
|
|
{
|
|
name: "settings changed and custom quality"
|
|
when: Cura.SimpleModeSettingsManager.isProfileCustomized && Cura.MachineManager.hasCustomQuality
|
|
PropertyChanges
|
|
{
|
|
target: warning
|
|
text: {
|
|
var profile_name = Cura.MachineManager.activeQualityChangesGroup.name
|
|
return catalog.i18nc("@info, %1 is the name of the custom profile", "<b>%1</b> custom profile is active and you overwrote some settings.").arg(profile_name)
|
|
}
|
|
}
|
|
|
|
},
|
|
State
|
|
{
|
|
name: "custom quality"
|
|
when: Cura.MachineManager.hasCustomQuality
|
|
PropertyChanges
|
|
{
|
|
target: warning
|
|
text: {
|
|
var profile_name = Cura.MachineManager.activeQualityChangesGroup.name
|
|
return catalog.i18nc("@info, %1 is the name of the custom profile", "<b>%1</b> custom profile is overriding some settings.").arg(profile_name)
|
|
}
|
|
}
|
|
},
|
|
State
|
|
{
|
|
name: "settings changed"
|
|
when: Cura.SimpleModeSettingsManager.isProfileCustomized
|
|
PropertyChanges
|
|
{
|
|
target: warning
|
|
text: catalog.i18nc("@info", "Some settings were changed.")
|
|
}
|
|
}
|
|
]
|
|
|
|
}
|
|
|
|
UM.SimpleButton
|
|
{
|
|
id: resetToDefaultQualityButton
|
|
height: UM.Theme.getSize("action_button_icon").height
|
|
width: height
|
|
iconSource: UM.Theme.getIcon("ArrowReset")
|
|
anchors
|
|
{
|
|
right: parent.right
|
|
verticalCenter: parent.verticalCenter
|
|
}
|
|
|
|
color: UM.Theme.getColor("accent_1")
|
|
|
|
onClicked:
|
|
{
|
|
Cura.MachineManager.resetToUseDefaultQuality()
|
|
}
|
|
}
|
|
|
|
} |