mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-03 10:25:10 +08:00
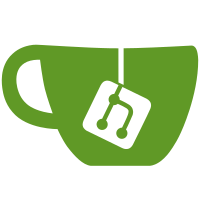
I was hoping to completely nix the generic materials model (since it's basically just a brand "Generic", but then in the QML it has to be have the same in terms of sub-menus or fold-outs and that looked stupid (Generic -> ABS -> ABS)). So we keep that one for now. It is cleaner though. Contributes to CURA-5162, CURA-5378
130 lines
5.1 KiB
Python
130 lines
5.1 KiB
Python
# Copyright (c) 2018 Ultimaker B.V.
|
|
# Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
from PyQt5.QtCore import Qt, pyqtSignal, pyqtProperty
|
|
from UM.Qt.ListModel import ListModel
|
|
from UM.Logger import Logger
|
|
from cura.Machines.Models.BaseMaterialsModel import BaseMaterialsModel
|
|
|
|
class MaterialTypesModel(ListModel):
|
|
|
|
def __init__(self, parent = None):
|
|
super().__init__(parent)
|
|
|
|
self.addRoleName(Qt.UserRole + 1, "name")
|
|
self.addRoleName(Qt.UserRole + 2, "colors")
|
|
|
|
class MaterialBrandsModel(BaseMaterialsModel):
|
|
|
|
extruderPositionChanged = pyqtSignal()
|
|
|
|
def __init__(self, parent = None):
|
|
super().__init__(parent)
|
|
|
|
from cura.CuraApplication import CuraApplication
|
|
|
|
self.addRoleName(Qt.UserRole + 1, "name")
|
|
self.addRoleName(Qt.UserRole + 2, "material_types")
|
|
|
|
self._container_registry = CuraApplication.getInstance().getContainerRegistry()
|
|
|
|
self._update()
|
|
|
|
def _update(self):
|
|
|
|
# Perform standard check and reset if the check fails
|
|
if not self._canUpdate():
|
|
self.setItems([])
|
|
return
|
|
|
|
# Get updated list of favorites
|
|
self._favorite_ids = self._material_manager.getFavorites()
|
|
|
|
brand_item_list = []
|
|
brand_group_dict = {}
|
|
|
|
# Part 1: Generate the entire tree of brands -> material types -> spcific materials
|
|
for root_material_id, container_node in self._available_materials.items():
|
|
metadata = container_node.metadata
|
|
|
|
# Do not include the materials from a to-be-removed package
|
|
if bool(metadata.get("removed", False)):
|
|
continue
|
|
|
|
# Add brands we haven't seen yet to the dict, skipping generics
|
|
brand = metadata["brand"]
|
|
if brand.lower() == "generic":
|
|
continue
|
|
if brand not in brand_group_dict:
|
|
brand_group_dict[brand] = {}
|
|
|
|
# Add material types we haven't seen yet to the dict
|
|
material_type = metadata["material"]
|
|
if material_type not in brand_group_dict[brand]:
|
|
brand_group_dict[brand][material_type] = []
|
|
|
|
# Now handle the individual materials
|
|
item = {
|
|
"root_material_id": root_material_id,
|
|
"id": metadata["id"],
|
|
"container_id": metadata["id"], # TODO: Remove duplicate in material manager qml
|
|
"GUID": metadata["GUID"],
|
|
"name": metadata["name"],
|
|
"brand": metadata["brand"],
|
|
"description": metadata["description"],
|
|
"material": metadata["material"],
|
|
"color_name": metadata["color_name"],
|
|
"color_code": metadata["color_code"],
|
|
"density": metadata.get("properties", {}).get("density", ""),
|
|
"diameter": metadata.get("properties", {}).get("diameter", ""),
|
|
"approximate_diameter": metadata["approximate_diameter"],
|
|
"adhesion_info": metadata["adhesion_info"],
|
|
"is_read_only": self._container_registry.isReadOnly(metadata["id"]),
|
|
"container_node": container_node,
|
|
"is_favorite": root_material_id in self._favorite_ids
|
|
}
|
|
brand_group_dict[brand][material_type].append(item)
|
|
|
|
# Part 2: Organize the tree into models
|
|
#
|
|
# Normally, the structure of the menu looks like this:
|
|
# Brand -> Material Type -> Specific Material
|
|
#
|
|
# To illustrate, a branded material menu may look like this:
|
|
# Ultimaker ┳ PLA ┳ Yellow PLA
|
|
# ┃ ┣ Black PLA
|
|
# ┃ ┗ ...
|
|
# ┃
|
|
# ┗ ABS ┳ White ABS
|
|
# ┗ ...
|
|
for brand, material_dict in brand_group_dict.items():
|
|
|
|
material_type_item_list = []
|
|
brand_item = {
|
|
"name": brand,
|
|
"material_types": MaterialTypesModel(self)
|
|
}
|
|
|
|
for material_type, material_list in material_dict.items():
|
|
material_type_item = {
|
|
"name": material_type,
|
|
"colors": BaseMaterialsModel(self)
|
|
}
|
|
material_type_item["colors"].clear()
|
|
|
|
# Sort materials by name
|
|
material_list = sorted(material_list, key = lambda x: x["name"].upper())
|
|
material_type_item["colors"].setItems(material_list)
|
|
|
|
material_type_item_list.append(material_type_item)
|
|
|
|
# Sort material type by name
|
|
material_type_item_list = sorted(material_type_item_list, key = lambda x: x["name"].upper())
|
|
brand_item["material_types"].setItems(material_type_item_list)
|
|
|
|
brand_item_list.append(brand_item)
|
|
|
|
# Sort brand by name
|
|
brand_item_list = sorted(brand_item_list, key = lambda x: x["name"].upper())
|
|
self.setItems(brand_item_list)
|