mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-05-02 16:54:23 +08:00
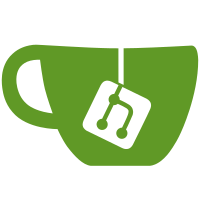
The MaterialManager.materialsUpdated signal was only called once upon init and for the rest when a favourite was added or removed. So only the FavoriteMaterialsModel would need to listen to it. Because the MaterialManager is being deprecated, the favourite materials model now just listens to the preferences changing instead, as it was supposed to be doing anyway. Contributes to issue CURA-6600.
47 lines
1.7 KiB
Python
47 lines
1.7 KiB
Python
# Copyright (c) 2019 Ultimaker B.V.
|
|
# Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
from cura.Machines.Models.BaseMaterialsModel import BaseMaterialsModel
|
|
import cura.CuraApplication # To listen to changes to the preferences.
|
|
|
|
## Model that shows the list of favorite materials.
|
|
class FavoriteMaterialsModel(BaseMaterialsModel):
|
|
def __init__(self, parent = None):
|
|
super().__init__(parent)
|
|
cura.CuraApplication.CuraApplication.getInstance().getPreferences().preferenceChanged.connect(self._onFavoritesChanged)
|
|
self._update()
|
|
|
|
## Triggered when any preference changes, but only handles it when the list
|
|
# of favourites is changed.
|
|
def _onFavoritesChanged(self, preference_key: str) -> None:
|
|
if preference_key != "cura/favorite_materials":
|
|
return
|
|
self._update()
|
|
|
|
def _update(self):
|
|
if not self._canUpdate():
|
|
return
|
|
|
|
# Get updated list of favorites
|
|
self._favorite_ids = self._material_manager.getFavorites()
|
|
|
|
item_list = []
|
|
|
|
for root_material_id, container_node in self._available_materials.items():
|
|
# Do not include the materials from a to-be-removed package
|
|
if bool(container_node.getMetaDataEntry("removed", False)):
|
|
continue
|
|
|
|
# Only add results for favorite materials
|
|
if root_material_id not in self._favorite_ids:
|
|
continue
|
|
|
|
item = self._createMaterialItem(root_material_id, container_node)
|
|
if item:
|
|
item_list.append(item)
|
|
|
|
# Sort the item list alphabetically by name
|
|
item_list = sorted(item_list, key = lambda d: d["brand"].upper())
|
|
|
|
self.setItems(item_list)
|