mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-16 06:01:49 +08:00
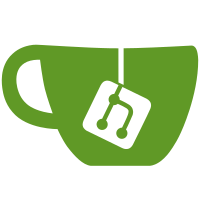
Conflicts: plugins/ImageReader/ConfigUI.qml plugins/PerObjectSettingsTool/PerObjectSettingsPanel.qml plugins/PerObjectSettingsTool/SettingPickDialog.qml resources/qml/Dialogs/DiscardOrKeepProfileChangesDialog.qml resources/qml/Menus/ConfigurationMenu/CustomConfiguration.qml resources/qml/Preferences/GeneralPage.qml resources/qml/Preferences/Materials/MaterialsPage.qml resources/qml/Preferences/Materials/MaterialsView.qml resources/qml/Preferences/ProfilesPage.qml These conflicts are all arising from headers/includes being updated at the same time, or from the two branches marking the other one's components as needing OldControls. This introduced more OldControls markers which don't get marked as merge conflicts by Git. This happens when an element could just be left as the original name but from the new import (e.g. a Button stays a Button in Controls 2, but should be marked as from OldControls on the branch that doesn't update the Button).
134 lines
3.5 KiB
QML
134 lines
3.5 KiB
QML
//Copyright (c) 2022 Ultimaker B.V.
|
|
//Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
import QtQuick 2.2
|
|
import QtQuick.Controls 2.2
|
|
|
|
import UM 1.5 as UM
|
|
import Cura 1.0 as Cura
|
|
import ".."
|
|
|
|
UM.Dialog
|
|
{
|
|
id: settingPickDialog
|
|
|
|
title: catalog.i18nc("@title:window", "Select Settings to Customize for this model")
|
|
width: screenScaleFactor * 360
|
|
|
|
property var additional_excluded_settings
|
|
|
|
onVisibilityChanged:
|
|
{
|
|
// force updating the model to sync it with addedSettingsModel
|
|
if (visible)
|
|
{
|
|
listview.model.forceUpdate()
|
|
updateFilter()
|
|
}
|
|
}
|
|
|
|
function updateFilter()
|
|
{
|
|
var new_filter = {}
|
|
new_filter["settable_per_mesh"] = true
|
|
// Don't filter on "settable_per_meshgroup" any more when `printSequencePropertyProvider.properties.value`
|
|
// is set to "one_at_a_time", because the current backend architecture isn't ready for that.
|
|
|
|
if (filterInput.text != "")
|
|
{
|
|
new_filter["i18n_label"] = "*" + filterInput.text
|
|
}
|
|
|
|
listview.model.filter = new_filter
|
|
}
|
|
|
|
TextField
|
|
{
|
|
id: filterInput
|
|
selectByMouse: true
|
|
|
|
anchors
|
|
{
|
|
top: parent.top
|
|
left: parent.left
|
|
right: toggleShowAll.left
|
|
rightMargin: UM.Theme.getSize("default_margin").width
|
|
}
|
|
|
|
placeholderText: catalog.i18nc("@label:textbox", "Filter...")
|
|
|
|
onTextChanged: settingPickDialog.updateFilter()
|
|
}
|
|
|
|
CheckBox
|
|
{
|
|
id: toggleShowAll
|
|
anchors
|
|
{
|
|
top: parent.top
|
|
right: parent.right
|
|
}
|
|
text: catalog.i18nc("@label:checkbox", "Show all")
|
|
}
|
|
|
|
ListView
|
|
{
|
|
id: listview
|
|
anchors
|
|
{
|
|
top: filterInput.bottom
|
|
left: parent.left
|
|
right: parent.right
|
|
bottom: parent.bottom
|
|
}
|
|
|
|
ScrollBar.vertical: UM.ScrollBar {}
|
|
clip: true
|
|
|
|
model: UM.SettingDefinitionsModel
|
|
{
|
|
id: definitionsModel
|
|
containerId: Cura.MachineManager.activeMachine != null ? Cura.MachineManager.activeMachine.definition.id: ""
|
|
visibilityHandler: UM.SettingPreferenceVisibilityHandler {}
|
|
expanded: [ "*" ]
|
|
exclude:
|
|
{
|
|
var excluded_settings = [ "machine_settings", "command_line_settings", "support_mesh", "anti_overhang_mesh", "cutting_mesh", "infill_mesh" ]
|
|
excluded_settings = excluded_settings.concat(settingPickDialog.additional_excluded_settings)
|
|
return excluded_settings
|
|
}
|
|
showAll: toggleShowAll.checked || filterInput.text !== ""
|
|
}
|
|
delegate: Loader
|
|
{
|
|
id: loader
|
|
|
|
width: listview.width
|
|
height: model.type != undefined ? UM.Theme.getSize("section").height : 0
|
|
|
|
property var definition: model
|
|
property var settingDefinitionsModel: definitionsModel
|
|
|
|
asynchronous: true
|
|
source:
|
|
{
|
|
switch(model.type)
|
|
{
|
|
case "category":
|
|
return "PerObjectCategory.qml"
|
|
default:
|
|
return "PerObjectItem.qml"
|
|
}
|
|
}
|
|
}
|
|
Component.onCompleted: settingPickDialog.updateFilter()
|
|
}
|
|
|
|
rightButtons: [
|
|
Button
|
|
{
|
|
text: catalog.i18nc("@action:button", "Close")
|
|
onClicked: settingPickDialog.visible = false
|
|
}
|
|
]
|
|
} |