mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-15 07:01:50 +08:00
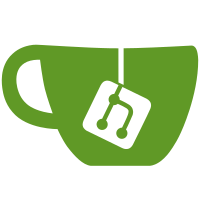
Introduced a new property 'show_in_menu' to control the visibility of individual settings in 3MFWriter plugin. Modified 'SettingExport' class constructor to store this visibility status. Also, updated the 'SettingsExportGroup' model to filter the settings based on visibility before presenting them in the GUI. CURA-11723
59 lines
1.6 KiB
Python
59 lines
1.6 KiB
Python
# Copyright (c) 2024 Ultimaker B.V.
|
|
# Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
from enum import IntEnum
|
|
|
|
from PyQt6.QtCore import QObject, pyqtProperty, pyqtEnum
|
|
|
|
|
|
class SettingsExportGroup(QObject):
|
|
|
|
@pyqtEnum
|
|
class Category(IntEnum):
|
|
Global = 0
|
|
Extruder = 1
|
|
Model = 2
|
|
|
|
def __init__(self, stack, name, category, settings, category_details = '', extruder_index = 0, extruder_color = ''):
|
|
super().__init__()
|
|
self.stack = stack
|
|
self._name = name
|
|
self._settings = settings
|
|
self._category = category
|
|
self._category_details = category_details
|
|
self._extruder_index = extruder_index
|
|
self._extruder_color = extruder_color
|
|
self._visible_settings = []
|
|
|
|
@pyqtProperty(str, constant=True)
|
|
def name(self):
|
|
return self._name
|
|
|
|
@pyqtProperty(list, constant=True)
|
|
def settings(self):
|
|
return self._settings
|
|
|
|
@pyqtProperty(list, constant=True)
|
|
def visibleSettings(self):
|
|
if self._visible_settings == []:
|
|
for item in self._settings:
|
|
if item.isVisible:
|
|
self._visible_settings.append(item)
|
|
return self._visible_settings
|
|
|
|
@pyqtProperty(int, constant=True)
|
|
def category(self):
|
|
return self._category
|
|
|
|
@pyqtProperty(str, constant=True)
|
|
def category_details(self):
|
|
return self._category_details
|
|
|
|
@pyqtProperty(int, constant=True)
|
|
def extruder_index(self):
|
|
return self._extruder_index
|
|
|
|
@pyqtProperty(str, constant=True)
|
|
def extruder_color(self):
|
|
return self._extruder_color
|