mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-05-03 17:24:21 +08:00
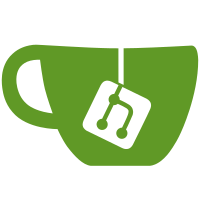
Since we now always send setting values as string and the conversion from float to string already does the rounding, there is no need to also do it here. Contributes to CURA-1278 Contributes to CURA-389
120 lines
3.6 KiB
QML
120 lines
3.6 KiB
QML
// Copyright (c) 2015 Ultimaker B.V.
|
|
// Uranium is released under the terms of the AGPLv3 or higher.
|
|
|
|
import QtQuick 2.2
|
|
import QtQuick.Controls 1.2
|
|
|
|
import UM 1.1 as UM
|
|
|
|
SettingItem
|
|
{
|
|
id: base
|
|
|
|
contents: Rectangle
|
|
{
|
|
id: control
|
|
|
|
anchors.fill: parent
|
|
|
|
border.width: UM.Theme.getSize("default_lining").width
|
|
border.color: !enabled ? UM.Theme.getColor("setting_control_disabled_border") : hovered ? UM.Theme.getColor("setting_control_border_highlight") : UM.Theme.getColor("setting_control_border")
|
|
|
|
property variant parentValue: value //From parent loader
|
|
function notifyReset() {
|
|
input.text = format(parentValue)
|
|
}
|
|
|
|
color: {
|
|
if (!enabled)
|
|
{
|
|
return UM.Theme.getColor("setting_control_disabled")
|
|
}
|
|
switch(propertyProvider.properties.validationState)
|
|
{
|
|
case "ValidatorState.Exception":
|
|
return UM.Theme.getColor("setting_validation_error")
|
|
case "ValidatorState.MinimumError":
|
|
return UM.Theme.getColor("setting_validation_error")
|
|
case "ValidatorState.MaximumError":
|
|
return UM.Theme.getColor("setting_validation_error")
|
|
case "ValidatorState.MinimumWarning":
|
|
return UM.Theme.getColor("setting_validation_warning")
|
|
case "ValidatorState.MaximumWarning":
|
|
return UM.Theme.getColor("setting_validation_warning")
|
|
case "ValidatorState.Valid":
|
|
return UM.Theme.getColor("setting_validation_ok")
|
|
|
|
default:
|
|
return UM.Theme.getColor("setting_control")
|
|
}
|
|
}
|
|
|
|
Rectangle
|
|
{
|
|
anchors.fill: parent;
|
|
anchors.margins: UM.Theme.getSize("default_lining").width;
|
|
color: UM.Theme.getColor("setting_control_highlight")
|
|
opacity: !control.hovered ? 0 : propertyProvider.properties.validationState == "ValidatorState.Valid" ? 1.0 : 0.35;
|
|
}
|
|
|
|
Label
|
|
{
|
|
anchors.right: parent.right;
|
|
anchors.rightMargin: UM.Theme.getSize("setting_unit_margin").width
|
|
anchors.verticalCenter: parent.verticalCenter;
|
|
|
|
text: definition.unit;
|
|
color: UM.Theme.getColor("setting_unit")
|
|
font: UM.Theme.getFont("default")
|
|
}
|
|
|
|
MouseArea
|
|
{
|
|
id: mouseArea
|
|
anchors.fill: parent;
|
|
//hoverEnabled: true;
|
|
cursorShape: Qt.IBeamCursor
|
|
}
|
|
|
|
TextInput
|
|
{
|
|
id: input
|
|
|
|
anchors
|
|
{
|
|
left: parent.left
|
|
leftMargin: UM.Theme.getSize("setting_unit_margin").width
|
|
right: parent.right
|
|
verticalCenter: parent.verticalCenter
|
|
}
|
|
|
|
Keys.onReleased:
|
|
{
|
|
propertyProvider.setPropertyValue("value", text)
|
|
}
|
|
|
|
onEditingFinished:
|
|
{
|
|
propertyProvider.setPropertyValue("value", text)
|
|
}
|
|
|
|
color: !enabled ? UM.Theme.getColor("setting_control_disabled_text") : UM.Theme.getColor("setting_control_text")
|
|
font: UM.Theme.getFont("default");
|
|
|
|
selectByMouse: true;
|
|
|
|
maximumLength: 10;
|
|
|
|
validator: RegExpValidator { regExp: /[0-9.,-]{0,10}/ }
|
|
|
|
Binding
|
|
{
|
|
target: input
|
|
property: "text"
|
|
value: propertyProvider.properties.value
|
|
when: !input.activeFocus
|
|
}
|
|
}
|
|
}
|
|
}
|