mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-05-03 01:04:35 +08:00
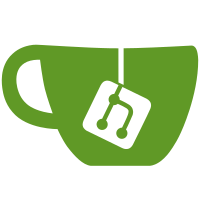
Contributes to CURA-1278 * settings_rework: (224 commits) Improve slice trigger documentation Import Cura in materials preferences page so we can use the active definition id Add layer height to high quality profile so we have something that changes Update example XML material to use the right product names Filter available materials by the machine definition Show the add machine dialog when we do not have an active machine Create machine-specific material containers for machine specific overrides in XML material files When creating a new container stack, add empty containers for things where we cannot find containers Add preferred variant, material and quality to UM2+ definition Account for global container stack being None in the backend plugin Use the global stack instance variable and account for it potentially being None Store the global container stack as an instance property Added wildcard to filtering Per object settings filter now uses correct bool types (instead of strings) Removed stray = sign. Fix creating print job name Disable asynchronous loading of SettingItem when Qt Version < 5.5 Document QTbug Properly serialise all settings to g-code file Document GCodeWriter class ...
277 lines
7.7 KiB
QML
277 lines
7.7 KiB
QML
// Copyright (c) 2015 Ultimaker B.V.
|
|
// Cura is released under the terms of the AGPLv3 or higher.
|
|
|
|
pragma Singleton
|
|
|
|
import QtQuick 2.2
|
|
import QtQuick.Controls 1.1
|
|
import UM 1.1 as UM
|
|
|
|
Item
|
|
{
|
|
property alias open: openAction;
|
|
property alias quit: quitAction;
|
|
|
|
property alias undo: undoAction;
|
|
property alias redo: redoAction;
|
|
|
|
property alias deleteSelection: deleteSelectionAction;
|
|
|
|
property alias deleteObject: deleteObjectAction;
|
|
property alias centerObject: centerObjectAction;
|
|
property alias groupObjects: groupObjectsAction;
|
|
property alias unGroupObjects:unGroupObjectsAction;
|
|
property alias mergeObjects: mergeObjectsAction;
|
|
//property alias unMergeObjects: unMergeObjectsAction;
|
|
|
|
property alias multiplyObject: multiplyObjectAction;
|
|
|
|
property alias deleteAll: deleteAllAction;
|
|
property alias reloadAll: reloadAllAction;
|
|
property alias resetAllTranslation: resetAllTranslationAction;
|
|
property alias resetAll: resetAllAction;
|
|
|
|
property alias addMachine: addMachineAction;
|
|
property alias configureMachines: settingsAction;
|
|
property alias addProfile: addProfileAction;
|
|
property alias updateProfile: updateProfileAction;
|
|
property alias resetProfile: resetProfileAction;
|
|
property alias manageProfiles: manageProfilesAction;
|
|
|
|
property alias preferences: preferencesAction;
|
|
|
|
property alias showEngineLog: showEngineLogAction;
|
|
property alias documentation: documentationAction;
|
|
property alias reportBug: reportBugAction;
|
|
property alias about: aboutAction;
|
|
|
|
property alias toggleFullScreen: toggleFullScreenAction;
|
|
|
|
property alias configureSettingVisibility: configureSettingVisibilityAction
|
|
|
|
UM.I18nCatalog{id: catalog; name:"cura"}
|
|
|
|
Action
|
|
{
|
|
id:toggleFullScreenAction
|
|
text: catalog.i18nc("@action:inmenu","Toggle Fu&ll Screen");
|
|
iconName: "view-fullscreen";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: undoAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","&Undo");
|
|
iconName: "edit-undo";
|
|
shortcut: StandardKey.Undo;
|
|
onTriggered: UM.OperationStack.undo();
|
|
enabled: UM.OperationStack.canUndo;
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: redoAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","&Redo");
|
|
iconName: "edit-redo";
|
|
shortcut: StandardKey.Redo;
|
|
onTriggered: UM.OperationStack.redo();
|
|
enabled: UM.OperationStack.canRedo;
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: quitAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:file","&Quit");
|
|
iconName: "application-exit";
|
|
shortcut: StandardKey.Quit;
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: preferencesAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:settings","&Preferences...");
|
|
iconName: "configure";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: addMachineAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:printer","&Add Printer...");
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: settingsAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:printer","Manage Pr&inters...");
|
|
iconName: "configure";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: updateProfileAction;
|
|
enabled: UM.ActiveProfile.valid && !UM.ActiveProfile.readOnly && UM.ActiveProfile.hasCustomisedValues
|
|
text: catalog.i18nc("@action:inmenu menubar:profile","&Update Current Profile");
|
|
onTriggered: UM.ActiveProfile.updateProfile();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: resetProfileAction;
|
|
enabled: UM.ActiveProfile.valid && UM.ActiveProfile.hasCustomisedValues
|
|
text: catalog.i18nc("@action:inmenu menubar:profile","&Reload Current Profile");
|
|
onTriggered: UM.ActiveProfile.discardChanges();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: addProfileAction;
|
|
enabled: UM.ActiveProfile.valid
|
|
text: catalog.i18nc("@action:inmenu menubar:profile","&Create New Profile...");
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: manageProfilesAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:profile","Manage Profiles...");
|
|
iconName: "configure";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: documentationAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:help","Show Online &Documentation");
|
|
iconName: "help-contents";
|
|
shortcut: StandardKey.Help;
|
|
onTriggered: CuraActions.openDocumentation();
|
|
}
|
|
|
|
Action {
|
|
id: reportBugAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:help","Report a &Bug");
|
|
iconName: "tools-report-bug";
|
|
onTriggered: CuraActions.openBugReportPage();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: aboutAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:help","&About...");
|
|
iconName: "help-about";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: deleteSelectionAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","Delete &Selection");
|
|
enabled: UM.Controller.toolsEnabled;
|
|
iconName: "edit-delete";
|
|
shortcut: StandardKey.Delete;
|
|
onTriggered: Printer.deleteSelection();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: deleteObjectAction;
|
|
text: catalog.i18nc("@action:inmenu","Delete Object");
|
|
enabled: UM.Controller.toolsEnabled;
|
|
iconName: "edit-delete";
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: centerObjectAction;
|
|
text: catalog.i18nc("@action:inmenu","Ce&nter Object on Platform");
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: groupObjectsAction
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","&Group Objects");
|
|
enabled: UM.Scene.numObjectsSelected > 1 ? true: false
|
|
iconName: "object-group"
|
|
shortcut: "Ctrl+G";
|
|
onTriggered: Printer.groupSelected();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: unGroupObjectsAction
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","Ungroup Objects");
|
|
enabled: UM.Scene.isGroupSelected
|
|
iconName: "object-ungroup"
|
|
shortcut: "Ctrl+Shift+G";
|
|
onTriggered: Printer.ungroupSelected();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: mergeObjectsAction
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","&Merge Objects");
|
|
enabled: UM.Scene.numObjectsSelected > 1 ? true: false
|
|
iconName: "merge";
|
|
shortcut: "Ctrl+Alt+G";
|
|
onTriggered: Printer.mergeSelected();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: multiplyObjectAction;
|
|
text: catalog.i18nc("@action:inmenu","&Duplicate Object");
|
|
iconName: "edit-duplicate"
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: deleteAllAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","&Clear Build Platform");
|
|
enabled: UM.Controller.toolsEnabled;
|
|
iconName: "edit-delete";
|
|
shortcut: "Ctrl+D";
|
|
onTriggered: Printer.deleteAll();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: reloadAllAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:file","Re&load All Objects");
|
|
iconName: "document-revert";
|
|
onTriggered: Printer.reloadAll();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: resetAllTranslationAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","Reset All Object Positions");
|
|
onTriggered: Printer.resetAllTranslation();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: resetAllAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:edit","Reset All Object &Transformations");
|
|
onTriggered: Printer.resetAll();
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: openAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:file","&Open File...");
|
|
iconName: "document-open";
|
|
shortcut: StandardKey.Open;
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: showEngineLogAction;
|
|
text: catalog.i18nc("@action:inmenu menubar:help","Show Engine &Log...");
|
|
iconName: "view-list-text";
|
|
shortcut: StandardKey.WhatsThis;
|
|
}
|
|
|
|
Action
|
|
{
|
|
id: configureSettingVisibilityAction
|
|
text: catalog.i18nc("@action:menu", "Configure setting visiblity...");
|
|
}
|
|
}
|