mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-13 18:21:48 +08:00
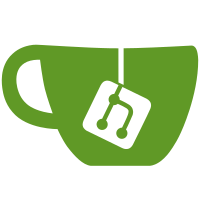
Removed the dependency of the DiscoveredCloudPrintersModel on CloudOutputDevice, which is Ultimaker specific. This can allow even external plugins to add to this model in the future. CURA-7022
61 lines
2.2 KiB
Python
61 lines
2.2 KiB
Python
from typing import Optional, TYPE_CHECKING, List, Dict
|
|
|
|
from PyQt5.QtCore import QObject, pyqtSlot, Qt, pyqtSignal, pyqtProperty
|
|
|
|
from UM.Qt.ListModel import ListModel
|
|
|
|
if TYPE_CHECKING:
|
|
from cura.CuraApplication import CuraApplication
|
|
|
|
|
|
class DiscoveredCloudPrintersModel(ListModel):
|
|
DeviceKeyRole = Qt.UserRole + 1
|
|
DeviceNameRole = Qt.UserRole + 2
|
|
DeviceTypeRole = Qt.UserRole + 3
|
|
DeviceFirmwareVersionRole = Qt.UserRole + 4
|
|
|
|
cloudPrintersDetectedChanged = pyqtSignal(bool)
|
|
|
|
def __init__(self, application: "CuraApplication", parent: Optional["QObject"] = None) -> None:
|
|
super().__init__(parent)
|
|
|
|
self.addRoleName(self.DeviceKeyRole, "key")
|
|
self.addRoleName(self.DeviceNameRole, "name")
|
|
self.addRoleName(self.DeviceTypeRole, "machine_type")
|
|
self.addRoleName(self.DeviceFirmwareVersionRole, "firmware_version")
|
|
|
|
self._discovered_ultimaker_cloud_printers_list = [] # type: List[Dict[str, str]]
|
|
self._new_cloud_printers_detected = False # type: bool
|
|
self._application = application # type: CuraApplication
|
|
|
|
def addDiscoveredCloudPrinters(self, new_devices: List[Dict[str, str]]) -> None:
|
|
self._discovered_ultimaker_cloud_printers_list.extend(new_devices)
|
|
self._update()
|
|
|
|
# Inform whether new cloud printers have been detected. If they have, the welcome wizard can close.
|
|
self._new_cloud_printers_detected = len(new_devices) > 0
|
|
self.cloudPrintersDetectedChanged.emit(len(new_devices) > 0)
|
|
|
|
@pyqtSlot()
|
|
def clear(self) -> None:
|
|
self._discovered_ultimaker_cloud_printers_list = []
|
|
self._update()
|
|
self._new_cloud_printers_detected = False
|
|
self.cloudPrintersDetectedChanged.emit(False)
|
|
|
|
def _update(self) -> None:
|
|
items = []
|
|
|
|
for cloud_printer in self._discovered_ultimaker_cloud_printers_list:
|
|
items.append(cloud_printer)
|
|
|
|
# Execute all filters.
|
|
filtered_items = list(items)
|
|
|
|
filtered_items.sort(key = lambda k: k["name"])
|
|
self.setItems(filtered_items)
|
|
|
|
@pyqtProperty(bool, notify = cloudPrintersDetectedChanged)
|
|
def newCloudPrintersDetected(self) -> bool:
|
|
return self._new_cloud_printers_detected
|