mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-04-22 21:59:37 +08:00
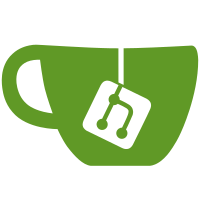
The local printer list utilizes the whole space, but the network printer list is only use half of the space.
153 lines
4.4 KiB
QML
153 lines
4.4 KiB
QML
// Copyright (c) 2019 Ultimaker B.V.
|
|
// Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
import QtQuick 2.10
|
|
import QtQuick.Controls 2.3
|
|
|
|
import UM 1.3 as UM
|
|
import Cura 1.1 as Cura
|
|
|
|
|
|
//
|
|
// This component contains the content for the "Add a printer" (network) page of the welcome on-boarding process.
|
|
//
|
|
Item
|
|
{
|
|
UM.I18nCatalog { id: catalog; name: "cura" }
|
|
|
|
Label
|
|
{
|
|
id: titleLabel
|
|
anchors.top: parent.top
|
|
anchors.horizontalCenter: parent.horizontalCenter
|
|
horizontalAlignment: Text.AlignHCenter
|
|
text: catalog.i18nc("@label", "Add a printer")
|
|
color: UM.Theme.getColor("primary_button")
|
|
font: UM.Theme.getFont("huge")
|
|
renderType: Text.NativeRendering
|
|
}
|
|
|
|
DropDownWidget
|
|
{
|
|
id: addNetworkPrinterDropDown
|
|
|
|
anchors.top: titleLabel.bottom
|
|
anchors.left: parent.left
|
|
anchors.right: parent.right
|
|
anchors.topMargin: UM.Theme.getSize("wide_margin").height
|
|
|
|
title: catalog.i18nc("@label", "Add a networked printer")
|
|
contentShown: true // by default expand the network printer list
|
|
|
|
onClicked:
|
|
{
|
|
if (contentShown)
|
|
{
|
|
addLocalPrinterDropDown.contentShown = false
|
|
}
|
|
}
|
|
|
|
contentComponent: networkPrinterListComponent
|
|
|
|
Component
|
|
{
|
|
id: networkPrinterListComponent
|
|
|
|
AddNetworkPrinterScrollView
|
|
{
|
|
id: networkPrinterScrollView
|
|
|
|
maxItemCountAtOnce: 10 // show at max 10 items at once, otherwise you need to scroll.
|
|
|
|
onRefreshButtonClicked:
|
|
{
|
|
UM.OutputDeviceManager.startDiscovery()
|
|
}
|
|
|
|
onAddByIpButtonClicked:
|
|
{
|
|
base.goToPage("add_printer_by_ip")
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
DropDownWidget
|
|
{
|
|
id: addLocalPrinterDropDown
|
|
|
|
anchors.top: addNetworkPrinterDropDown.bottom
|
|
anchors.left: parent.left
|
|
anchors.right: parent.right
|
|
anchors.topMargin: UM.Theme.getSize("wide_margin").height
|
|
|
|
title: catalog.i18nc("@label", "Add a non-networked printer")
|
|
|
|
onClicked:
|
|
{
|
|
if (contentShown)
|
|
{
|
|
addNetworkPrinterDropDown.contentShown = false
|
|
}
|
|
}
|
|
|
|
contentComponent: localPrinterListComponent
|
|
|
|
Component
|
|
{
|
|
id: localPrinterListComponent
|
|
|
|
AddLocalPrinterScrollView
|
|
{
|
|
id: localPrinterView
|
|
}
|
|
}
|
|
}
|
|
|
|
Cura.PrimaryButton
|
|
{
|
|
id: nextButton
|
|
anchors.right: parent.right
|
|
anchors.bottom: parent.bottom
|
|
enabled:
|
|
{
|
|
// If the network printer dropdown is expanded, make sure that there is a selected item
|
|
if (addNetworkPrinterDropDown.contentShown)
|
|
{
|
|
return addNetworkPrinterDropDown.contentItem.currentItem != null
|
|
}
|
|
else
|
|
{
|
|
// Printer name cannot be empty
|
|
const localPrinterItem = addLocalPrinterDropDown.contentItem.currentItem
|
|
const isPrinterNameValid = addLocalPrinterDropDown.contentItem.isPrinterNameValid
|
|
return localPrinterItem != null && isPrinterNameValid
|
|
}
|
|
}
|
|
|
|
text: base.currentItem.next_page_button_text
|
|
onClicked:
|
|
{
|
|
// Create a network printer or a local printer according to the selection
|
|
if (addNetworkPrinterDropDown.contentShown)
|
|
{
|
|
// Create a network printer
|
|
const networkPrinterItem = addNetworkPrinterDropDown.contentItem.currentItem
|
|
CuraApplication.getDiscoveredPrintersModel().createMachineFromDiscoveredPrinter(networkPrinterItem)
|
|
|
|
// If we have created a machine, go to the last page, which is the "cloud" page.
|
|
base.goToPage("cloud")
|
|
}
|
|
else
|
|
{
|
|
// Create a local printer
|
|
const localPrinterItem = addLocalPrinterDropDown.contentItem.currentItem
|
|
const printerName = addLocalPrinterDropDown.contentItem.printerName
|
|
Cura.MachineManager.addMachine(localPrinterItem.id, printerName)
|
|
|
|
base.showNextPage()
|
|
}
|
|
}
|
|
}
|
|
}
|