mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-12 17:01:46 +08:00
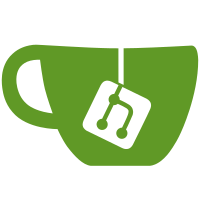
* 'master' of github.com:ultimaker/Cura: (98 commits) 15.10 Restyling of the message stack Fixed machine head polygons Removed unused settings from fdmprinter Added action for togling fullscreen Fixed usage of wrong checkbox in viewpage Added preference to disable automatic push free Added preference to disable auto center when selecting object Cleanup 15.10 Restyling toolbar, viewmode button Update README.md Update README.md Added rudementary 3 point bed leveling 15.10 New font for Cura 15.10 Changes the styling for the open file button 15.10 Changes the label names for the different action buttons Fixed typo Endstop now stops listening upon destruction 15.10 Re-alignment of the messagestack Cleaned up code a bit Added heated bed check ...
90 lines
2.7 KiB
Python
90 lines
2.7 KiB
Python
# Copyright (c) 2015 Ultimaker B.V.
|
|
# Cura is released under the terms of the AGPLv3 or higher.
|
|
|
|
from UM.Scene.SceneNode import SceneNode
|
|
from UM.Resources import Resources
|
|
from UM.Math.Color import Color
|
|
from UM.Math.Vector import Vector
|
|
from UM.Mesh.MeshData import MeshData
|
|
|
|
import numpy
|
|
|
|
class ConvexHullNode(SceneNode):
|
|
def __init__(self, node, hull, parent = None):
|
|
super().__init__(parent)
|
|
|
|
self.setCalculateBoundingBox(False)
|
|
|
|
self._material = None
|
|
|
|
self._original_parent = parent
|
|
|
|
self._inherit_orientation = False
|
|
self._inherit_scale = False
|
|
|
|
self._color = Color(35, 35, 35, 0.5)
|
|
|
|
self._node = node
|
|
self._node.transformationChanged.connect(self._onNodePositionChanged)
|
|
self._node.parentChanged.connect(self._onNodeParentChanged)
|
|
self._node.decoratorsChanged.connect(self._onNodeDecoratorsChanged)
|
|
self._onNodeDecoratorsChanged(self._node)
|
|
|
|
self._hull = hull
|
|
|
|
hull_points = self._hull.getPoints()
|
|
mesh = MeshData()
|
|
if len(hull_points) > 3:
|
|
center = (hull_points.min(0) + hull_points.max(0)) / 2.0
|
|
mesh.addVertex(center[0], 0.1, center[1])
|
|
else: #Hull has not enough points
|
|
return
|
|
for point in hull_points:
|
|
mesh.addVertex(point[0], 0.1, point[1])
|
|
indices = []
|
|
for i in range(len(hull_points) - 1):
|
|
indices.append([0, i + 1, i + 2])
|
|
|
|
indices.append([0, mesh.getVertexCount() - 1, 1])
|
|
|
|
mesh.addIndices(numpy.array(indices, numpy.int32))
|
|
|
|
self.setMeshData(mesh)
|
|
|
|
def getWatchedNode(self):
|
|
return self._node
|
|
|
|
def render(self, renderer):
|
|
if not self._material:
|
|
self._material = renderer.createMaterial(Resources.getPath(Resources.Shaders, "basic.vert"), Resources.getPath(Resources.Shaders, "color.frag"))
|
|
|
|
if self.getParent():
|
|
self._material.setUniformValue("u_color", self._color)
|
|
renderer.queueNode(self, material = self._material, transparent = True)
|
|
|
|
return True
|
|
|
|
def _onNodePositionChanged(self, node):
|
|
if node.callDecoration("getConvexHull"):
|
|
node.callDecoration("setConvexHull", None)
|
|
node.callDecoration("setConvexHullNode", None)
|
|
self.setParent(None)
|
|
|
|
def _onNodeParentChanged(self, node):
|
|
if node.getParent():
|
|
self.setParent(self._original_parent)
|
|
else:
|
|
self.setParent(None)
|
|
|
|
def _onNodeDecoratorsChanged(self, node):
|
|
self._color = Color(35, 35, 35, 0.5)
|
|
|
|
if not node:
|
|
return
|
|
|
|
if node.hasDecoration("getProfile"):
|
|
self._color.setR(0.75)
|
|
|
|
if node.hasDecoration("getSetting"):
|
|
self._color.setG(0.75)
|