mirror of
https://git.mirrors.martin98.com/https://github.com/google/draco
synced 2025-07-04 17:15:10 +08:00
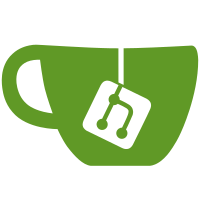
1. Add 'use strict'to example Javascript. 2. Small fixes to README.md. 3. Fix Draco compiler flag tests. 4. Fixed issue when number of quantized bits was to large. 5. Changed StartDecoding to return a value. 6. Check that num of attributes are not less than 0. 7. Check that the number of attributes is a valid value.
123 lines
3.4 KiB
HTML
123 lines
3.4 KiB
HTML
<html>
|
|
<head>
|
|
<title>Draco Javascript Decode Timing</title>
|
|
|
|
<script type="text/javascript" src="draco_decoder.js"> </script>
|
|
|
|
<script type="text/javascript">
|
|
'use strict';
|
|
|
|
// String to hold table output.
|
|
let dt = '';
|
|
|
|
function startTable() {
|
|
dt += '<table><tr>';
|
|
dt += '<td>Filename</td>';
|
|
dt += '<td>Total milli</td>';
|
|
dt += '<td>Decode milli</td>';
|
|
dt += '<td>Size bytes</td>';
|
|
dt += '<td>Num points</td>';
|
|
}
|
|
|
|
function addCell(str, newRow) {
|
|
if (newRow)
|
|
dt += '</tr><tr>';
|
|
dt += '<td>' + str + '</td>';
|
|
}
|
|
|
|
function finishTable() {
|
|
dt += '</table>';
|
|
document.getElementById('tableOutput').innerHTML = dt;
|
|
}
|
|
|
|
function onDecodeClick() {
|
|
startTable();
|
|
const inputs = document.getElementById('u').value.split(',');
|
|
s_log('Decoding ' + inputs.length + ' files...', true, true);
|
|
TestMeshDecodingAsync(inputs, 0);
|
|
}
|
|
|
|
function onDecodeMultipleClick() {
|
|
startTable();
|
|
const inputs = document.getElementById('u').value.split(',');
|
|
const decode_count = parseInt(document.getElementById('decode_count').value);
|
|
s_log('Decoding ' + (decode_count * inputs.length) + ' files...', true, true);
|
|
|
|
let fileList = [];
|
|
for (let i = 0; i < decode_count; ++i) {
|
|
fileList = fileList.concat(inputs);
|
|
}
|
|
TestMeshDecodingAsync(fileList, 0);
|
|
}
|
|
|
|
function TestMeshDecodingAsync(filenameList, index) {
|
|
const xhr = new XMLHttpRequest();
|
|
xhr.open("GET", filenameList[index], true);
|
|
xhr.responseType = "arraybuffer";
|
|
|
|
xhr.onload = function(event) {
|
|
const arrayBuffer = xhr.response;
|
|
if (arrayBuffer) {
|
|
const byteArray = new Uint8Array(arrayBuffer);
|
|
|
|
const total_t0 = performance.now();
|
|
|
|
const buffer = new Module.DecoderBuffer();
|
|
buffer.Init(byteArray, byteArray.length);
|
|
|
|
const wrapper = new Module.WebIDLWrapper();
|
|
|
|
const decode_t0 = performance.now();
|
|
const geometryType = wrapper.GetEncodedGeometryType(buffer);
|
|
let outputGeometry;
|
|
if (geometryType == Module.TRIANGULAR_MESH) {
|
|
outputGeometry = wrapper.DecodeMeshFromBuffer(buffer);
|
|
} else {
|
|
outputGeometry = wrapper.DecodePointCloudFromBuffer(buffer);
|
|
}
|
|
const t1 = performance.now();
|
|
|
|
addCell(filenameList[index], true);
|
|
addCell('' + (t1 - total_t0), false);
|
|
addCell('' + (t1 - decode_t0), false);
|
|
addCell('' + byteArray.length, false);
|
|
addCell('' + outputGeometry.num_points(), false);
|
|
|
|
Module.destroy(outputGeometry);
|
|
Module.destroy(wrapper);
|
|
Module.destroy(buffer);
|
|
|
|
if (index < filenameList.length - 1) {
|
|
index = index + 1;
|
|
TestMeshDecodingAsync(filenameList, index);
|
|
} else {
|
|
finishTable();
|
|
}
|
|
}
|
|
};
|
|
|
|
xhr.send(null);
|
|
}
|
|
|
|
function s_log(str, end_line, reset) {
|
|
if (reset)
|
|
document.getElementById('status').innerHTML = '';
|
|
document.getElementById('status').innerHTML += str;
|
|
if (end_line)
|
|
document.getElementById('status').innerHTML += "<br/>";
|
|
}
|
|
|
|
</script>
|
|
</head>
|
|
<body>
|
|
<H1>Draco Javascript Decode Timing</H1>
|
|
Draco file to be decoded. If more than one file, add as comma separated list. E.g. "file1.drc,file2.drc,file3.drc"</br>
|
|
<input id="u" type="text" size="80" value="input.drc"/><input type="button" value="Decode" onClick="onDecodeClick();">
|
|
<input id="decode_count" type="text" size="10" value="10"/><input type="button" value="Decode Multiple" onClick="onDecodeMultipleClick();">
|
|
<br/>
|
|
<div id="status"> </div></br>
|
|
<div id="tableOutput"> </div>
|
|
</body>
|
|
</html>
|
|
|