mirror of
https://gitlab.com/libeigen/eigen.git
synced 2025-07-05 12:45:11 +08:00
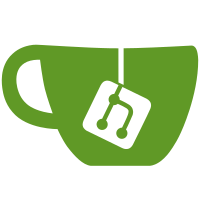
The added check `ctorleak.cpp` demonstrates how the leak can be reproduced. The test appears to pass but it is leaking the storage of the (not created) matrix. I don't know how to make this test fail in the existing test suite but you can run it through Valgrind (or another debugger) to verify the leak. $ ./check.sh ctorleak && valgrind --leak-check=full ./test/ctorleak This patch fixes this leak by adding some try-catch-delete-rethrow blocks to `Eigen/src/Core/util/Memory.h`.
44 lines
900 B
C++
44 lines
900 B
C++
#include "main.h"
|
|
|
|
#include <exception> // std::exception
|
|
|
|
struct Foo
|
|
{
|
|
int dummy;
|
|
Foo() { if (!internal::random(0, 10)) throw Foo::Fail(); }
|
|
class Fail : public std::exception {};
|
|
};
|
|
|
|
namespace Eigen
|
|
{
|
|
template<>
|
|
struct NumTraits<Foo>
|
|
{
|
|
typedef double Real;
|
|
typedef double NonInteger;
|
|
typedef double Nested;
|
|
enum
|
|
{
|
|
IsComplex = 0,
|
|
IsInteger = 1,
|
|
ReadCost = -1,
|
|
AddCost = -1,
|
|
MulCost = -1,
|
|
IsSigned = 1,
|
|
RequireInitialization = 1
|
|
};
|
|
static inline Real epsilon() { return 1.0; }
|
|
static inline Real dummy_epsilon() { return 0.0; }
|
|
};
|
|
}
|
|
|
|
void test_ctorleak()
|
|
{
|
|
try
|
|
{
|
|
Matrix<Foo, Dynamic, Dynamic> m(14, 92);
|
|
eigen_assert(false); // not reached
|
|
}
|
|
catch (const Foo::Fail&) { /* ignore */ }
|
|
}
|