mirror of
https://git.mirrors.martin98.com/https://github.com/Ultimaker/Cura
synced 2025-07-02 13:15:11 +08:00
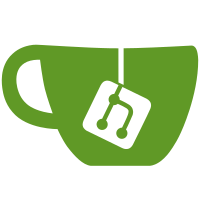
We created a new set of icons for Cura. These icons had to be reverted though because they weren't working out in the interface for the last release yet. This unreverts them, basically adding them back hoping that we'll get them fixed in time for the next release. Contributes to issue CURA-8342. Revert "Revert "Fix merge conflict"" This reverts commit bb20e3307f43edc1ff53cb154d2351ddfe39e158. Revert "Revert "Merge pull request #9716 from Ultimaker/CURA-8010_new_icons"" This reverts commit 70e4e9640e561e18a12870f30c905203ce8ccee7. Revert "Revert "Fix typo in icon name"" This reverts commit 38ce22ba7c3f40b971bc6e1e0a8e776ca9d51512. Revert "Revert "Add list for deprecated icons"" This reverts commit 119a957e7f978dbf1ddbcb3b0005bf38e8fed943. Revert "Revert "Add Function icon"" This reverts commit 760726cf0bb953bb1b0fc277b448f419d4bd2544. Revert "Revert "Switch out inherit icon"" This reverts commit 26afff609381e2004d194c280f504b6226859bd3. Revert "Revert "Merge branch 'CURA-8205_Introduce_new_icons_in_Cura' of github.com:Ultimaker/Cura"" This reverts commit 6483db3d47ee052c1a966cdee3af7190577a5769. Revert "Fix incorrect icons" This reverts commit 02a4ade2a50a943ff36fd4895bdc9261cf2133eb.
289 lines
10 KiB
QML
289 lines
10 KiB
QML
// Copyright (c) 2019 Ultimaker B.V.
|
|
// Cura is released under the terms of the LGPLv3 or higher.
|
|
|
|
import QtQuick 2.10
|
|
import QtQuick.Controls 2.3
|
|
|
|
import UM 1.3 as UM
|
|
import Cura 1.1 as Cura
|
|
|
|
|
|
//
|
|
// This is the scroll view widget for adding a (local) printer. This scroll view shows a list view with printers
|
|
// categorized into 3 categories: "Ultimaker", "Custom", and "Other".
|
|
//
|
|
Item
|
|
{
|
|
id: base
|
|
|
|
// The currently selected machine item in the local machine list.
|
|
property var currentItem: (machineList.currentIndex >= 0)
|
|
? machineList.model.getItem(machineList.currentIndex)
|
|
: null
|
|
// The currently active (expanded) section/category, where section/category is the grouping of local machine items.
|
|
property string currentSection: "Ultimaker B.V."
|
|
// By default (when this list shows up) we always expand the "Ultimaker" section.
|
|
property var preferredCategories: {
|
|
"Ultimaker B.V.": -2,
|
|
"Custom": -1
|
|
}
|
|
|
|
// User-editable printer name
|
|
property alias printerName: printerNameTextField.text
|
|
property alias isPrinterNameValid: printerNameTextField.acceptableInput
|
|
|
|
onCurrentItemChanged:
|
|
{
|
|
printerName = currentItem == null ? "" : currentItem.name
|
|
}
|
|
|
|
function updateCurrentItemUponSectionChange()
|
|
{
|
|
// Find the first machine from this section
|
|
for (var i = 0; i < machineList.count; i++)
|
|
{
|
|
var item = machineList.model.getItem(i)
|
|
if (item.section == base.currentSection)
|
|
{
|
|
machineList.currentIndex = i
|
|
break
|
|
}
|
|
}
|
|
}
|
|
|
|
function getMachineName()
|
|
{
|
|
return machineList.model.getItem(machineList.currentIndex) != undefined ? machineList.model.getItem(machineList.currentIndex).name : "";
|
|
}
|
|
|
|
function getMachineMetaDataEntry(key)
|
|
{
|
|
var metadata = machineList.model.getItem(machineList.currentIndex) != undefined ? machineList.model.getItem(machineList.currentIndex).metadata : undefined;
|
|
if (metadata)
|
|
{
|
|
return metadata[key];
|
|
}
|
|
return undefined;
|
|
}
|
|
|
|
Component.onCompleted:
|
|
{
|
|
updateCurrentItemUponSectionChange()
|
|
}
|
|
|
|
Row
|
|
{
|
|
id: localPrinterSelectionItem
|
|
anchors.left: parent.left
|
|
anchors.right: parent.right
|
|
anchors.top: parent.top
|
|
|
|
// ScrollView + ListView for selecting a local printer to add
|
|
Cura.ScrollView
|
|
{
|
|
id: scrollView
|
|
|
|
height: childrenHeight
|
|
width: Math.floor(parent.width * 0.48)
|
|
|
|
ListView
|
|
{
|
|
id: machineList
|
|
|
|
// CURA-6793
|
|
// Enabling the buffer seems to cause the blank items issue. When buffer is enabled, if the ListView's
|
|
// individual item has a dynamic change on its visibility, the ListView doesn't redraw itself.
|
|
// The default value of cacheBuffer is platform-dependent, so we explicitly disable it here.
|
|
cacheBuffer: 0
|
|
boundsBehavior: Flickable.StopAtBounds
|
|
flickDeceleration: 20000 // To prevent the flicking behavior.
|
|
model: UM.DefinitionContainersModel
|
|
{
|
|
id: machineDefinitionsModel
|
|
filter: { "visible": true }
|
|
sectionProperty: "manufacturer"
|
|
preferredSections: preferredCategories
|
|
}
|
|
|
|
section.property: "section"
|
|
section.delegate: sectionHeader
|
|
delegate: machineButton
|
|
}
|
|
|
|
Component
|
|
{
|
|
id: sectionHeader
|
|
|
|
Button
|
|
{
|
|
id: button
|
|
width: ListView.view.width
|
|
height: UM.Theme.getSize("action_button").height
|
|
text: section
|
|
|
|
property bool isActive: base.currentSection == section
|
|
|
|
background: Rectangle
|
|
{
|
|
anchors.fill: parent
|
|
color: isActive ? UM.Theme.getColor("setting_control_highlight") : "transparent"
|
|
}
|
|
|
|
contentItem: Item
|
|
{
|
|
width: childrenRect.width
|
|
height: UM.Theme.getSize("action_button").height
|
|
|
|
UM.RecolorImage
|
|
{
|
|
id: arrow
|
|
anchors.left: parent.left
|
|
width: UM.Theme.getSize("standard_arrow").width
|
|
height: UM.Theme.getSize("standard_arrow").height
|
|
sourceSize.width: width
|
|
sourceSize.height: height
|
|
color: UM.Theme.getColor("text")
|
|
source: base.currentSection == section ? UM.Theme.getIcon("ChevronSingleDown") : UM.Theme.getIcon("ChevronSingleRight")
|
|
}
|
|
|
|
Label
|
|
{
|
|
id: label
|
|
anchors.left: arrow.right
|
|
anchors.leftMargin: UM.Theme.getSize("default_margin").width
|
|
verticalAlignment: Text.AlignVCenter
|
|
text: button.text
|
|
font: UM.Theme.getFont("default_bold")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
}
|
|
}
|
|
|
|
onClicked:
|
|
{
|
|
base.currentSection = section
|
|
base.updateCurrentItemUponSectionChange()
|
|
}
|
|
}
|
|
}
|
|
|
|
Component
|
|
{
|
|
id: machineButton
|
|
|
|
Cura.RadioButton
|
|
{
|
|
id: radioButton
|
|
anchors.left: parent.left
|
|
anchors.leftMargin: UM.Theme.getSize("standard_list_lineheight").width
|
|
anchors.right: parent.right
|
|
anchors.rightMargin: UM.Theme.getSize("default_margin").width
|
|
height: visible ? UM.Theme.getSize("standard_list_lineheight").height : 0
|
|
|
|
checked: ListView.view.currentIndex == index
|
|
text: name
|
|
visible: base.currentSection == section
|
|
onClicked: ListView.view.currentIndex = index
|
|
}
|
|
}
|
|
}
|
|
|
|
// Vertical line
|
|
Rectangle
|
|
{
|
|
id: verticalLine
|
|
anchors.top: parent.top
|
|
height: childrenHeight - UM.Theme.getSize("default_lining").height
|
|
width: UM.Theme.getSize("default_lining").height
|
|
color: UM.Theme.getColor("lining")
|
|
}
|
|
|
|
// User-editable printer name row
|
|
Column
|
|
{
|
|
width: Math.floor(parent.width * 0.52)
|
|
|
|
spacing: UM.Theme.getSize("default_margin").width
|
|
padding: UM.Theme.getSize("default_margin").width
|
|
|
|
Label
|
|
{
|
|
width: parent.width - (2 * UM.Theme.getSize("default_margin").width)
|
|
wrapMode: Text.Wrap
|
|
text: base.getMachineName()
|
|
color: UM.Theme.getColor("primary_button")
|
|
font: UM.Theme.getFont("huge")
|
|
elide: Text.ElideRight
|
|
}
|
|
Grid
|
|
{
|
|
width: parent.width
|
|
columns: 2
|
|
rowSpacing: UM.Theme.getSize("default_lining").height
|
|
columnSpacing: UM.Theme.getSize("default_margin").width
|
|
|
|
verticalItemAlignment: Grid.AlignVCenter
|
|
|
|
Label
|
|
{
|
|
id: manufacturerLabel
|
|
text: catalog.i18nc("@label", "Manufacturer")
|
|
font: UM.Theme.getFont("default")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
}
|
|
Label
|
|
{
|
|
text: base.getMachineMetaDataEntry("manufacturer")
|
|
width: parent.width - manufacturerLabel.width
|
|
font: UM.Theme.getFont("default")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
wrapMode: Text.WordWrap
|
|
}
|
|
Label
|
|
{
|
|
id: profileAuthorLabel
|
|
text: catalog.i18nc("@label", "Profile author")
|
|
font: UM.Theme.getFont("default")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
}
|
|
Label
|
|
{
|
|
text: base.getMachineMetaDataEntry("author")
|
|
width: parent.width - profileAuthorLabel.width
|
|
font: UM.Theme.getFont("default")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
wrapMode: Text.WordWrap
|
|
}
|
|
|
|
Label
|
|
{
|
|
id: printerNameLabel
|
|
text: catalog.i18nc("@label", "Printer name")
|
|
font: UM.Theme.getFont("default")
|
|
color: UM.Theme.getColor("text")
|
|
renderType: Text.NativeRendering
|
|
}
|
|
|
|
Cura.TextField
|
|
{
|
|
id: printerNameTextField
|
|
placeholderText: catalog.i18nc("@text", "Please name your printer")
|
|
maximumLength: 40
|
|
width: parent.width - (printerNameLabel.width + (3 * UM.Theme.getSize("default_margin").width))
|
|
validator: RegExpValidator
|
|
{
|
|
regExp: printerNameTextField.machineNameValidator.machineNameRegex
|
|
}
|
|
property var machineNameValidator: Cura.MachineNameValidator { }
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
}
|
|
}
|